Express.js - Handling POST Requests with Body Data
In this tutorial, we will see how to handle POST requests with body data in Express.js and setting up a form, parsing form data and sending responses. POST requests are commonly used to submit data to a server, such as through a form or API.
Set Up the Express.js Server
Create a file index.js inside the my-app folder and add the following code.
index.js
const express = require('express'); const app = express(); // Middleware to parse URL-encoded data app.use(express.urlencoded({ extended: true })); // Route to serve a simple form app.get('/', (req, res) => { res.send(` <form method="POST" action="/submit"> <input type="text" name="name" placeholder="Enter your name" required> <button type="submit">Submit</button> </form> `); }); // Handle POST request at /submit app.post('/submit', (req, res) => { const name = req.body.name; // Accessing the name field from the request body res.send(`Hello, ${name}!`); }); const PORT = 5000; app.listen(PORT, () => { console.log(`Server is running at http://localhost:${PORT}`); });
Run the Server
Run the server using the command given below.
node index.js
D:\my-app>node index.js Server is running on http://localhost:5000
Output
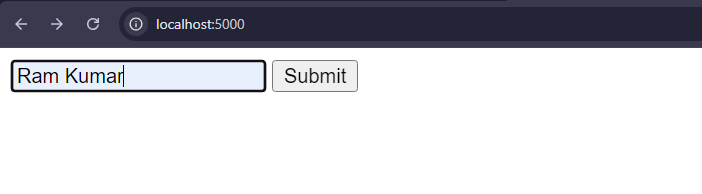
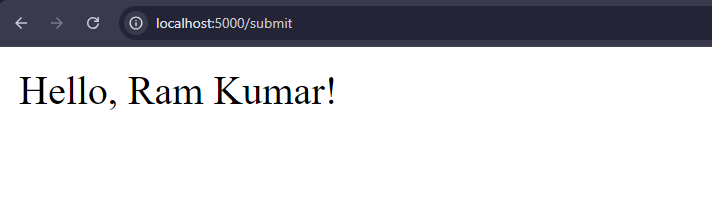