Express.js - Handlebars (HBS) Template Engine
Handlebars is a popular template engine for the Node.js web application framework Express. It allows you to generate dynamic HTML pages by combining templates with data in an efficient way. Handlebars is an extension of the Mustache templating language, and it allows you to embed expressions and logic in your HTML templates.
Key Features of Handlebars
- Expressions : It allows embedding data directly into HTML using {{}} syntax.
- Partials : Reusable template components like headers, footers and navigation bars.
- Layouts : Layouts provide a base structure for templates and is best practice for the DRY (Don't Repeat Yourself) principle.
- Helpers : Built-in Helpers are used to perform logic within templates.
Using Handlebars with Express.js
In an Express.js application, Handlebars serves as a view engine that takes template files ( .handlebars or .hbs ) and dynamically generates HTML content based on the provided data.
Install Express.js and Handlebars
Install express.js and handlebars using the command is given below.
npm install express hbs
D:\my-app>npm install express hbs added 9 packages, and audited 75 packages in 3s 14 packages are looking for funding run `npm fund` for details found 0 vulnerabilities
Create views Folder
Inside the my-app directory, create a views folder.
mkdir views
Create index.hbs File
Create an index.hbs file inside the views folder.
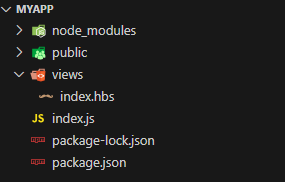
Set Up Express.js with Handlebars
Set Express to use Handlebars as the template engine.
const express = require('express'); const path = require('path'); const hbs = require('hbs'); const app = express(); // Set up handlebars as the view engine app.set('view engine', 'hbs'); // Define the location for your views app.set('views', path.join(__dirname, '/views')); // Define a Routes app.get('/', (req, res) => { res.render('index', { title: 'Welcome', message: 'Hello, Handlebars!' }); }); const PORT = 5000; app.listen(PORT, () => { console.log(`Server is running at http://localhost:${PORT}`); });
<!DOCTYPE html> <html lang="en"> <head> <title>{{title}}</title> </head> <body> <h1>{{message}}</h1> </body> </html>
Output
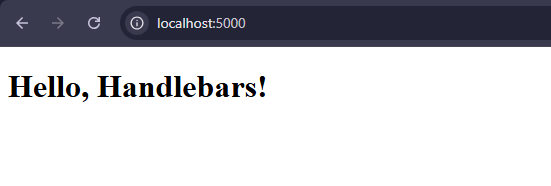