Express.js - Layout (hbs template)
What is Layout?
Layout refers to a template structure that is used across multiple pages of your web application. Instead of repeating the same HTML structure (like the header, footer, or sidebar) in every view, a layout allows you to define a common template for your pages.
Key Features of Layout
- Code Reusability : Layouts allow sharing common UI components across multiple pages.
- Centralized Structure : Keeps layout-related code in one place for better organization.
- Dynamic Content Injection : Allows pages to insert specific content while keeping the structure intact.
- Consistency : Ensures all pages follow the same design and layout standards.
- Improved Maintainability : Simplifies updates by modifying only the layout instead of multiple files.
- Flexible Structure : Supports dynamic adjustments without breaking the overall design.
- Support for Multiple Layouts : Enables different layouts for different sections of the app.
File and Folder Structure
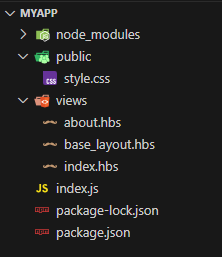
Setting up Express.js Server
This Express.js app serves static files, sets up Handlebars as the view engine with a layout, and dynamically renders the home and about page.
const express = require('express'); const path = require('path'); const hbs = require('hbs'); const app = express(); // Serve static files from the public directory app.use(express.static(path.join(__dirname, 'public'))); // Set up handlebars as the view engine app.set('view engine', 'hbs'); // Set the location of the views app.set('views', path.join(__dirname, 'views')); // If you want to set the layout for all routes //app.set('view options', { layout: 'base_layout' }); // Define a route app.get('/', (req, res) => { res.render('index', { title: 'Index Page', layout: "base_layout" }); }); app.get('/about', (req, res) => { res.render('about', { title: 'About Page', layout: "base_layout" }); }); const PORT = 5000; app.listen(PORT, () => { console.log(`Server is running on http://localhost:${PORT}`); });
Explanation of Configuration Lines
- app.set('views', path.join(__dirname, 'views')); : This line sets the directory where Express should look for the view templates (Handlebars files).
- app.set('view options', { layout: 'base_layout' }); : This line sets a base layout for the views in the Express app.
Create hbs Templates
i) Create base layout File
This Handlebars layout defines a structured HTML template with a header, navigation menu, main content area, and footer, where dynamic content is injected using {{{body}}}
<html> <head> <title>{{{title}}}</title> <link rel="stylesheet" href="/css/style.css"> </head> <body> <header> <div class="container"> <nav> <ul> <li><a href="/">Home</a></li> <li><a href="/about">About</a></li> <li><a href="/services">Services</a></li> <li><a href="/contact">Contact</a></li> </ul> </nav> </div> </header> <main> <div class="content-container"> {{{body}}} <!-- This is where the content from the views will be inserted --> </div> </main> <footer> <div class="footer-content"> <p>© 2025 All rights reserved.</p> <nav class="footer-nav"> <ul> <li><a href="/privacy-policy">Privacy Policy</a></li> <li><a href="/terms-of-service">Terms of Service</a></li> </ul> </nav> </div> </footer> </body> </html>
ii) Create Index File
This code displays a dynamic title inside an h2 tag and a welcome message inside a p tag on the index page.
<h2>{{{title}}}</h2> <p>Welcome to the index page</p>
ii) Create About File
This code displays a dynamic title inside an h2 tag and a welcome message inside a p tag on the about page.
<h2>{{{title}}}</h2> <p>Welcome to the about page</p>
iv) Create Style File
This CSS file defines global styles, a responsive layout, and consistent typography, ensuring a clean and structured design. It styles the header, navigation, main content, and footer, with hover effects and spacing for better user experience.
/* Global styles */ * { margin: 0; padding: 0; box-sizing: border-box; font-family: Arial, sans-serif; } /* Body and content */ body { background-color: #f4f4f4; color: #333; line-height: 1.6; } /* Container for overall layout */ .container { width: 90%; margin: 0 auto; } /* Header styles */ header { background-color: #2c3e50; padding: 20px 0; color: #fff; } .site-title { text-align: center; font-size: 2.5rem; margin-bottom: 10px; } nav ul { display: flex; justify-content: center; list-style-type: none; } nav ul li { margin: 0 15px; } nav ul li a { text-decoration: none; color: #ecf0f1; font-size: 1.1rem; transition: color 0.3s; } nav ul li a:hover { color: #3498db; } /* Main content styles */ main { padding: 40px 0; background-color: #fff; min-height: 60vh; } .content-container { max-width: 900px; margin: 0 auto; } /* Footer styles */ footer { background-color: #34495e; color: #fff; padding: 20px 0; text-align: center; } footer nav ul { display: flex; justify-content: center; list-style-type: none; } footer nav ul li { margin: 0 15px; } footer nav ul li a { text-decoration: none; color: #ecf0f1; font-size: 1rem; transition: color 0.3s; } footer nav ul li a:hover { color: #3498db; }
Run the Server
Run the server using the command is given below.
node index.js
D:\my-app>node index.js Server is running on http://localhost:5000
Output
Index Page
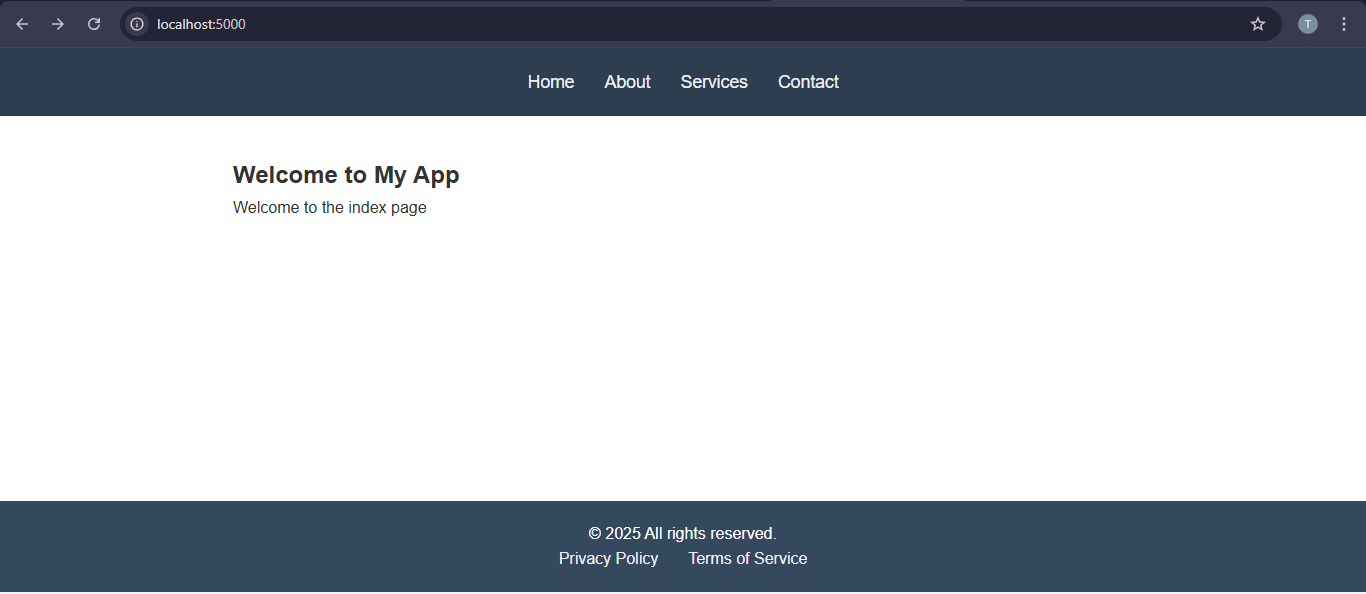
About Page
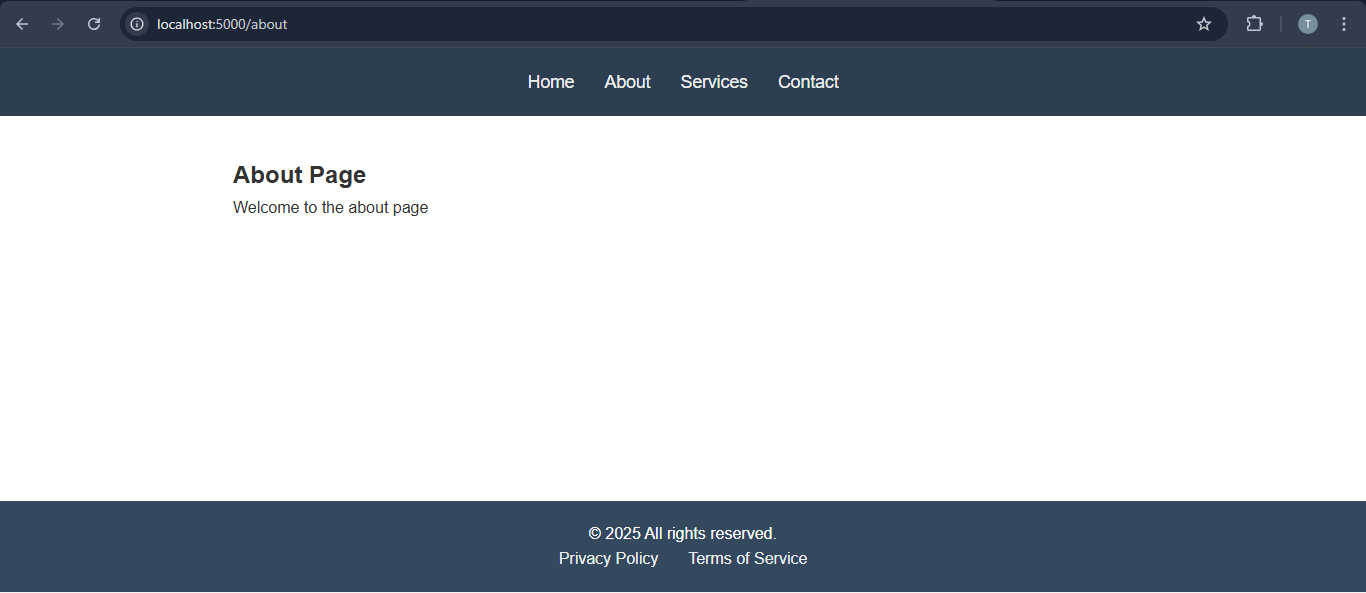