Express.js - Serving Static Files
A static file is any file (such as images, CSS files, JavaScript, and other media files) that the server sends to the client without modification. In Express.js, we can serve static files using the built-in express.static middleware. Here’s how you do it:
1. Set up a new Express project
Run the following commands to create a new Express project. This creates a package.json file
D:>mkdir my-app D:>cd my-app D:\my-app>npm init -y Wrote to D:\my-app\package.json: { "name": "my-app", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "keywords": [], "author": "", "license": "ISC" }
2. Install Express and Handlebars
Install Express and Handlebars in the project, use below command.
npm install express hbs
D:\my-app>npm install express hbs added 9 packages, and audited 75 packages in 3s 14 packages are looking for funding run `npm fund` for details found 0 vulnerabilities
3. Create the public and views Directory
Now, create the public and views directory inside my-app directory.
D:\my-app>mkdir public D:\my-app>cd public D:\my-app>mkdir views D:\my-app>cd views
Files and Folder Structure
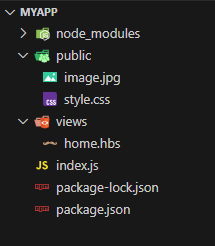
- The /public directory contains image.jpg and style.css.
- The /views directory contains Handlebars templates(home.hbs).
4. Setting Up an Express.js Server
Set up your Express application to serve static files and use Handlebars for templates.
index.jsconst express = require('express'); const path = require('path'); const hbs = require('hbs'); const app = express(); // Set up handlebars as the view engine app.set('view engine', 'hbs'); // Define the location for your views app.set('views', path.join(__dirname, '/views')); // Serve static files from the "public" folder app.use(express.static(path.join(__dirname, "public"))); // Define a route app.get('/', (req, res) => { res.render('index'); }); const PORT = 5000; app.listen(PORT, () => { console.log(`Server is running on http://localhost:${PORT}`); });
5. Create Handlebars Template
Inside your /views folder, create a home.hbs file.
views/home.hbs<html> <head> <title>Serve Static File</title> <link rel="stylesheet" href="/style.css"> <!-- Link to CSS --> </head> <body> <div class="container"> <img src="image.jpg"> </div> </body> </html>
6. Create style sheet
Inside your /public folder, create a style.css file. Here you can add the style of your html page.
public/style.css/* Center everything */ body { font-family: Arial, sans-serif; background-color: #f4f4f4; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; } /* Container for styling */ .container { text-align: center; background: white; padding: 20px; border-radius: 10px; box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.1); } /* image */ img { width: 500px; border-radius: 10px; box-shadow: 0px 4px 8px rgba(0, 0, 0, 0.2); }
Run the Server
Run the server using the command is given below.
node index.js
D:\my-app>node index.js Server is running on http://localhost:5000
Output
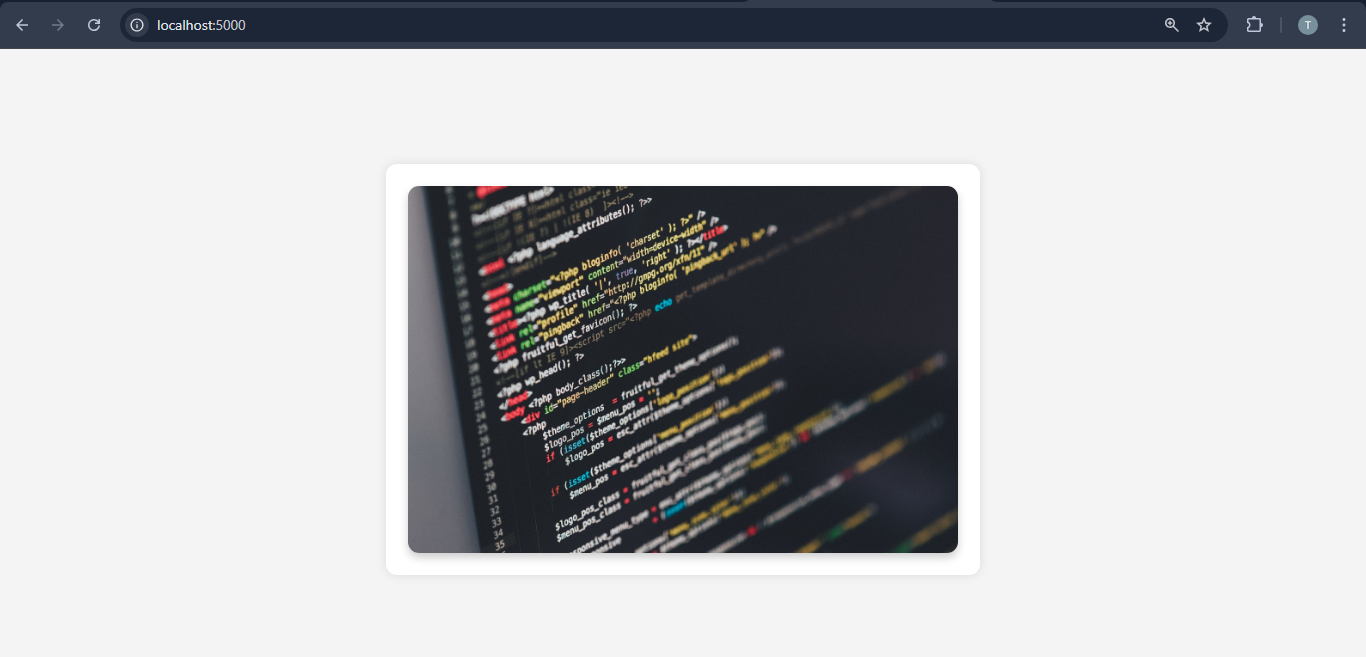